In this tutorial, I will show you how to navigate through Lightning Components using Lightning Web Components. In the GIF below you can see the final result.
![Navigate through Lightning Components [LWC] Navigate through Lightning Components [LWC]](https://www.sfwiki.de/wp-content/uploads/2021/04/navigate_through_components_lwc.gif)
<template>
<div class="slds-card slds-m-horizontal_large" style="font-family: 'Open Sans', sans-serif">
<!-- Spinner -->
<template if:true={showSpinner}>
<div class="slds-is-relative slds-m-around_large slds-align_absolute-center" style="min-height: 300px; !important;">
<lightning-spinner alternative-text="Loading..." variant="brand"></lightning-spinner>
</div>
</template>
<template if:false={showSpinner}>
<!-- Header -->
<div class="slds-card__header slds-p-bottom_small">
<header>
<lightning-progress-indicator current-step={step} type="path" variant="base" has-error="false">
<lightning-progress-step label="First Page" value="1" onclick={nextPage}></lightning-progress-step>
<lightning-progress-step label="Second Page" value="2" onclick={nextPage}></lightning-progress-step>
<lightning-progress-step label="Third Page" value="3" onclick={nextPage}></lightning-progress-step>
</lightning-progress-indicator>
</header>
</div>
<!-- First Page -->
<template if:true={showFirstPage}>
<div class="slds-scrollable_y" style="max-height: 600px; !important;">
<lightning-datatable key-field="Id"
data={listOfAccounts}
columns={accountColumns}
show-row-number-column="true"
>
</lightning-datatable>
</div>
</template>
<!-- Second Page -->
<template if:true={showSecondPage}>
<div class="slds-p-around_small">
<div class="slds-text-align_center slds-container_center" style="font-size: 15px;">
<p>This is the second Page</p>
<p>Hope you like this tutorial</p>
<p style="font-weight: bold; color: red">Sf <span style="font-weight: bold; color: black">Wiki</span></p>
</div>
</div>
</template>
<!-- Third Page -->
<template if:true={showThirdPage}>
<lightning-datatable key-field="Id"
data={listOfContacts}
columns={contactColumns}
show-row-number-column="true"
>
</lightning-datatable>
</template>
<!-- Footer -->
<footer class="slds-modal__footer slds-align_absolute-center" style="padding: 0.50rem 1rem;">
<template if:true={showFirstPage}>
<lightning-button label="Next" variant="neutral" value="2" onclick={nextPage}></lightning-button>
</template>
<template if:true={showSecondPage}>
<lightning-button class="slds-p-right_xx-small" label="Previous" variant="neutral" value="1" onclick={previousPage}></lightning-button>
<lightning-button label="Next" variant="neutral" value="3" onclick={nextPage}></lightning-button>
</template>
<template if:true={showThirdPage}>
<lightning-button class="slds-p-right_xx-small" label="Previous" variant="neutral" value="2" onclick={previousPage}></lightning-button>
<lightning-button label="Save" variant="brand"></lightning-button>
</template>
</footer>
</template>
</div>
</template>
import {LightningElement} from 'lwc';
//APEX-Methods
import getAccounts from '@salesforce/apex/SfWiki_Handler.getAccounts'
import getContacts from '@salesforce/apex/SfWiki_Handler.getContacts'
export default class LwcNavigateThroughComponents extends LightningElement {
step;
showSpinner;
//First Page
showFirstPage = true;
listOfAccounts;
accountColumns = [
{label: 'Name', fieldName: 'Name', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true},
{label: 'Phone', fieldName: 'Phone', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true},
{label: 'Website', fieldName: 'Website', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true},
{label: 'Industry', fieldName: 'Industry', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true},
{label: 'Annual Revenue', fieldName: 'AnnualRevenue', type: 'currency', sortable: false, wrapText: false, hideDefaultActions: true,
typeAttributes: {minimumFractionDigits: 2, maximumFractionDigits: 2},
cellAttributes: {alignment: "left"}}
];
//Second Page
showSecondPage;
//Third Page
showThirdPage;
listOfContacts;
contactColumns = [
{label: 'Name', fieldName: 'Name', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true},
{label: 'Title', fieldName: 'Title', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true},
{label: 'Phone', fieldName: 'Phone', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true},
{label: 'Email', fieldName: 'Email', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true},
{label: 'Department', fieldName: 'Department', type: 'text', sortable: true, wrapText: false, hideDefaultActions: true}
];
connectedCallback() {
this.getInitData();
}
getInitData() {
this.showSpinner = true;
getAccounts({})
.then(data => {
this.listOfAccounts = data;
this.showSpinner = false;
})
.catch(error => {
console.log(error);
});
}
nextPage(event) {
this.setAllStepsToFalse();
this.step = event.target.value;
if(this.step === "1") {
this.showFirstPage = true;
} else if(this.step === "2") {
this.showSecondPage = true;
} else if(this.step === "3") {
this.showSpinner = true;
getContacts({})
.then(data => {
this.listOfContacts = data;
this.showSpinner = false;
this.showThirdPage = true;
})
.catch(error => {
console.log(error);
});
}
}
previousPage(event) {
this.setAllStepsToFalse();
this.step = event.target.value;
if(this.step === "1") {
this.showFirstPage = true;
} else if(this.step === "2") {
this.showSecondPage = true;
}
}
setAllStepsToFalse() {
this.showFirstPage = false;
this.showSecondPage = false;
this.showThirdPage = false;
}
}
SfWiki_Handler.cls
public without sharing class SfWiki_Handler {
@AuraEnabled
public static List<Account> getAccounts() {
return [SELECT Id, Name, Phone, Website, Industry, AnnualRevenue FROM Account LIMIT 10];
}
@AuraEnabled
public static List<Contact> getContacts() {
return [SELECT Id, Name, Title, Phone, Email, Department FROM Contact LIMIT 10];
}
}
That’s it!
I hope you have enjoyed learning “How to navigate through Lightning Components using Lightning Web Components”. Please share your thoughts in the comments below. If you find value in this type of post, please subscribe because we have tons of tutorials in progress to be posted!
If you prefer to see the result with Lightning Aura Components, here’s the link to it: How to navigate through Lightning Components [Aura].
Leave a comment!
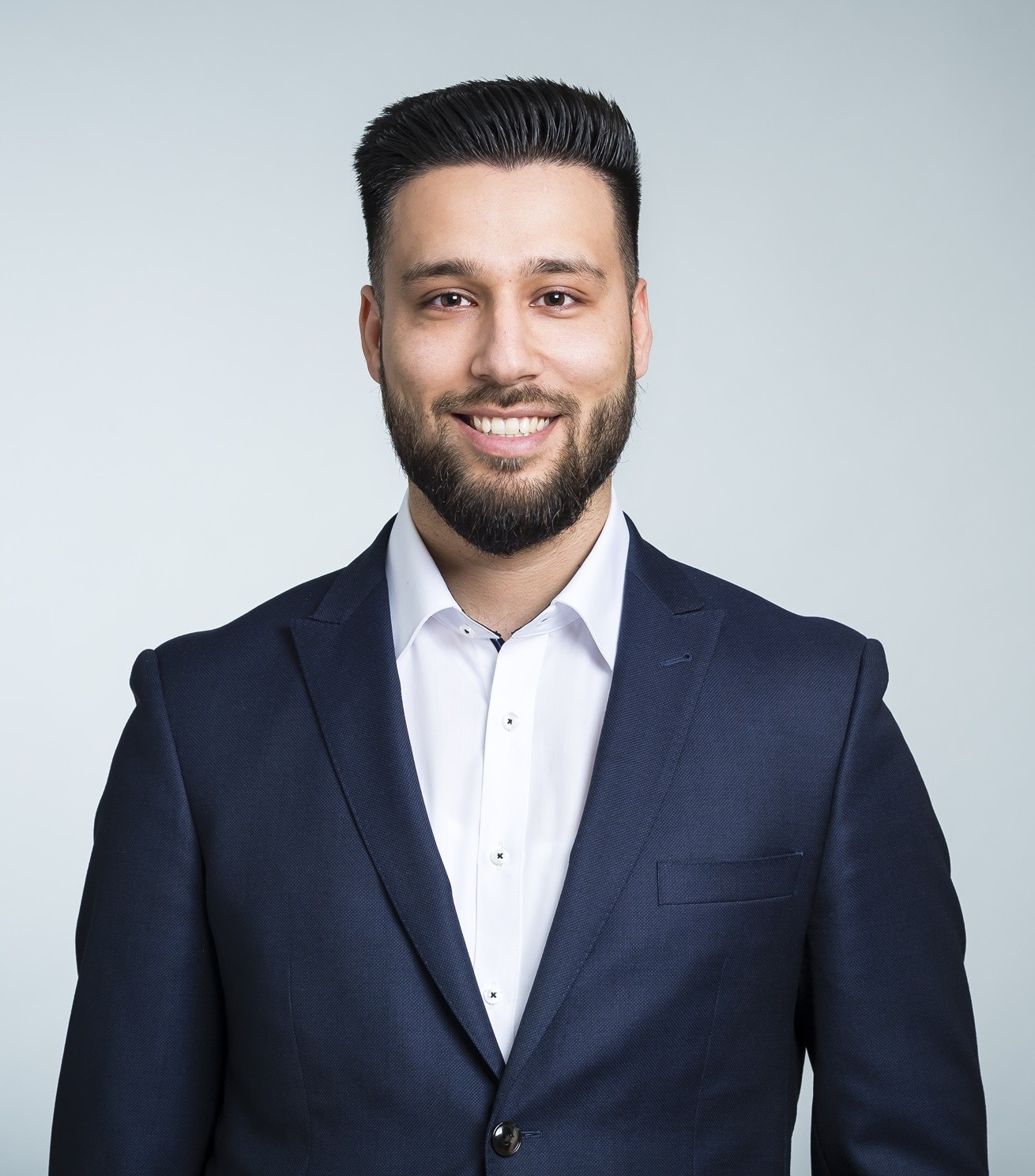
Hamid Abassi
SfWiki Founder
I am a Salesforce Developer from Hamburg who wants to help the Salesforce Community around the world with my tutorials. Feel free to explore my website and hopefully learn something new.